Postingan lainnya
Buku Ini Koding!
Baru!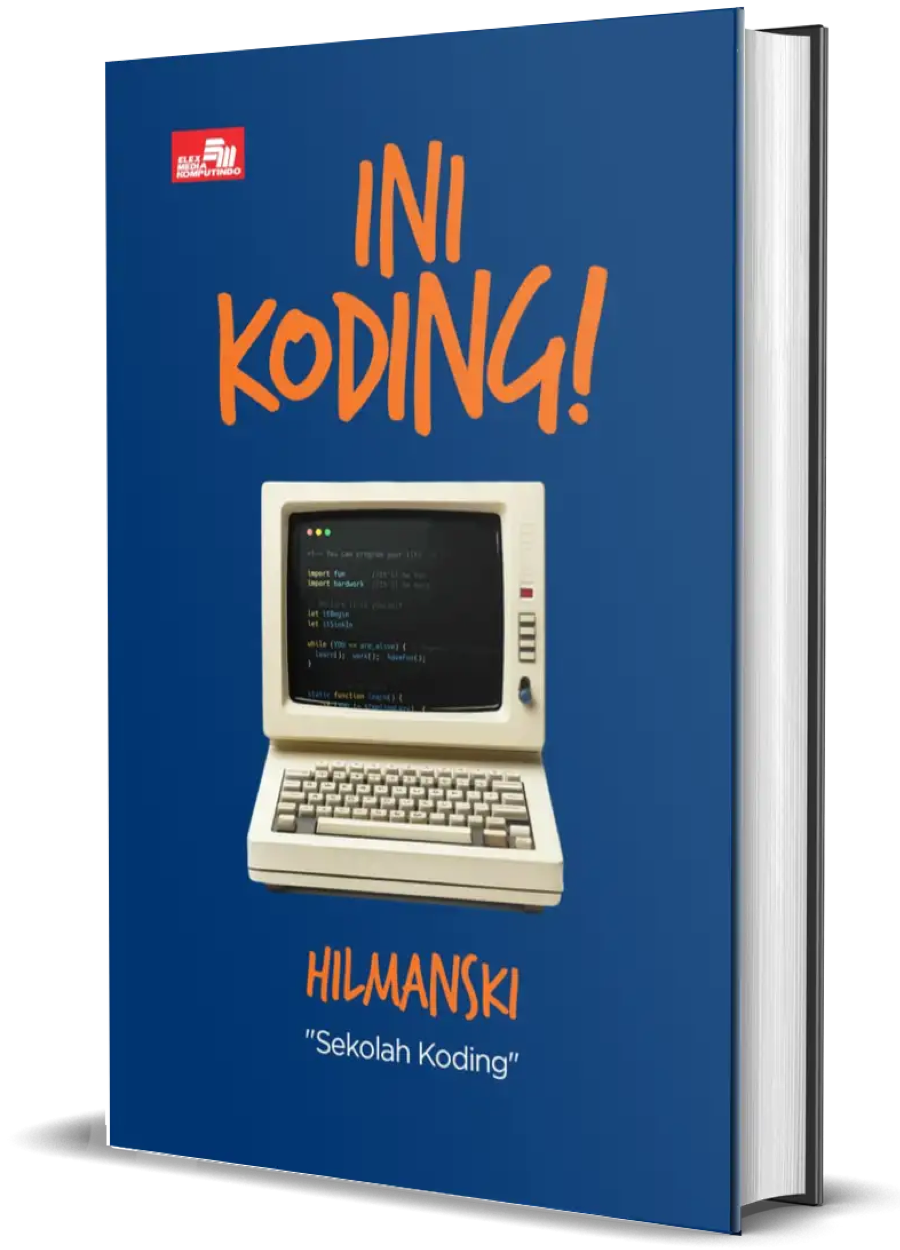
Buku ini akan jadi teman perjalanan kamu belajar sampai dapat kerjaan di dunia programming!
Memunculkan Gambar di data CRUD dari database
Saya Mencoba menampilkan gambar dari database namun tidak bisa,
Mohon Bantuannya
Controler :
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Admin extends CI_Controller {
public function __construct()
{
parent::__construct();
$this->load->library('form_validation');
}
/*
* Show Login page
*
*/
public function login()
{
$this->template->load('template','page/admin/login');
}
/*
* Process Login request method post
*
*/
public function auth()
{
$username = $this->input->post('username');
$password = $this->input->post('password');
# get user by username
$user = $this->db->get_where('admin_login',['username' => $username])->row();
# if user exists
if($user) {
#verify hash password
if(password_verify($password, $user->password)) {
# set session data
$data = [
'username' => $user->username,
'role' => 'admin'
];
$data = [
'username' => $user->username,
'role' => 'user'
];
$this->session->set_userdata($data);
redirect('admin/test');
} else {
alerterror('message','Password salah');
redirect('admin/login');
}
}
# user not found redirect to login page
else {
alerterror('message','Username tidak ditemukan');
redirect('admin/login');
}
}
public function test()
{
is_admin();
$this->db->order_by('nomor');
$data['soals'] = $this->db->get('test_online')->result();
$this->template->load('template','page/admin/test',$data);
}
public function soal_add()
{
is_admin();
if($this->form_validation->run('soal') == false) {
$this->template->load('template','page/admin/create_soal');
} else {
$data = [
'nomor' => $this->input->post('nomor'),
'soal' => $this->input->post('soal'),
'a' => $this->input->post('a'),
'b' => $this->input->post('b'),
'c' => $this->input->post('c'),
'jawaban' => $this->input->post('jawaban'),
'tanggal' => date('Y-m-d'),
];
$this->db->insert('test_online',$data);
alertsuccess('message','Data berhasil ditambahkan');
redirect('admin/test');
}
}
public function soal_edit($id)
{
is_admin();
if($this->form_validation->run('soal') == false) {
$data['soal'] = $this->db->get_where('test_online',['id' => $id])->row();
$this->template->load('template','page/admin/edit_soal',$data);
} else {
$data = [
'nomor' => $this->input->post('nomor'),
'soal' => $this->input->post('soal'),
'a' => $this->input->post('a'),
'b' => $this->input->post('b'),
'c' => $this->input->post('c'),
'jawaban' => $this->input->post('jawaban'),
'tanggal' => date('Y-m-d'),
];
$this->db->update('test_online',$data,['id' => $id]);
alertsuccess('message','Data berhasil diubah');
redirect('admin/test');
}
}
public function soal_del($id)
{
is_admin();
$this->db->delete('test_online',['id' => $id]);
alertsuccess('message','Data berhasil dihapus');
redirect('admin/test');
}
public function hasil_test()
{
is_admin();
$this->db->order_by('id','DESC');
$data['hasils'] = $this->db->get('hasil_test')->result();
$this->template->load('template','page/admin/hasil_test',$data);
}
public function cetak_hasil()
{
is_admin();
$data['hasils'] = $this->db->get('hasil_test')->result();
$this->load->view('page/admin/cetak_hasil',$data);
}
public function career()
{
is_admin();
$this->db->order_by('id','DESC');
$data['careers'] = $this->db->get('info_loker')->result();
$this->template->load('template','page/admin/career',$data);
}
public function career_add()
{
is_admin();
if($this->form_validation->run('career') == false) {
$this->template->load('template','page/admin/create_career');
} else {
$data = [
'info' => $this->input->post('info'),
'jabatan' => $this->input->post('jabatan'),
'bagian' => $this->input->post('bagian'),
'tanggal_akhir' => $this->input->post('tgl_akhir'),
'tanggal' => date('Y-m-d'),
];
$this->db->insert('info_loker',$data);
alertsuccess('message','Data berhasil ditambahkan');
redirect('admin/career');
}
}
public function career_edit($id)
{
is_admin();
if($this->form_validation->run('career') == false) {
$data['career'] = $this->db->get_where('info_loker',['id' => $id])->row();
$this->template->load('template','page/admin/edit_career',$data);
} else {
$data = [
'info' => $this->input->post('info'),
'jabatan' => $this->input->post('jabatan'),
'bagian' => $this->input->post('bagian'),
'tanggal_akhir' => $this->input->post('tgl_akhir'),
'tanggal' => date('Y-m-d'),
];
$this->db->update('info_loker',$data,['id' => $id]);
alertsuccess('message','Data berhasil diubah');
redirect('admin/career');
}
}
public function career_del($id)
{
is_admin();
$this->db->delete('info_loker',['id' => $id]);
alertsuccess('message','Data berhasil dihapus');
redirect('admin/career');
}
public function status()
{
is_admin();
$this->db->order_by('id','DESC');
$data['registrasis'] = $this->db->get('registrasi')->result();
$this->template->load('template','page/admin/status',$data);
}
public function status_add()
{
is_admin();
if($this->form_validation->run('status') == false) {
$this->template->load('template','page/admin/create_status');
} else {
$data = [
'nama_lengkap' => $this->input->post('nama_lengkap'),
'status_pengajuan' => $this->input->post('status_pengajuan'),
];
$this->db->insert('registrasi',$data);
alertsuccess('message','Data berhasil ditambahkan');
redirect('admin/status');
}
}
public function status_edit($id)
{
is_admin();
if($this->form_validation->run('registrasis') == false) {
$data['registrasis'] = $this->db->get_where('registrasi',['id' => $id])->row();
$this->template->load('template','page/admin/edit_status',$data);
} else {
$data = [
'nama_lengkap' => $this->input->post('nama_lengkap'),
'status_pengajuan' => $this->input->post('status_pengajuan'),
];
$this->db->update('registrasi',$data,['id' => $id]);
alertsuccess('message','Data berhasil diubah');
redirect('admin/status');
}
}
public function status_del($id)
{
is_admin();
$this->db->delete('registrasi',['id' => $id]);
alertsuccess('message','Data berhasil dihapus');
redirect('admin/status');
}
}
Status.Php
<div class="row mt-4">
<div class="col-md-14">
<div class="card">
<div class="card-header text-center">Status Pengajuan</div>
<div class="card-body">
<table class="table table-striped">
<thead>
<tr>
<th>Nama Lengkap</th>
<th>Foto Ijazah</th>
<th>Tanggal Lahir</th>
<th>Agama</th>
<th>Kewarganegaraan</th>
<th>Jenjang Pendidikan</th>
<th>Umur</th>
<th>Status</th>
<th>Jenis Kelamin</th>
<th>Alamat Lengkap</th>
<th>No Hp</th>
<th>Status Pengajuan</th>
</tr>
</thead>
<tbody>
<tr>
<td><?= $profil->nama_lengkap ?></td>
<Td>
<?php if($profil->pas_foto_ijazah != null) { ?>
<img src="<?base_url('assets/img/pelamar/' .$profil->pas_foto_ijazah)?>" style="width:100px">
<?php } ?>
</Td>
<td><?= $profil->tgl_lahir ?></td>
<td><?= $profil->agama ?></td>
<td><?= $profil->kewarganegaraan ?></td>
<td><?= $profil->jenjang_pendidikan ?></td>
<td><?= $profil->umur ?></td>
<td><?= $profil->status ?></td>
<td><?= $profil->jenis_kelamin ?></td>
<td><?= $profil->alamat_lengkap ?></td>
<td><?= $profil->no_hp ?></td>
<td><?= $profil->status_pengajuan ?></td>
</tr>
</table>
</div>
</div>
</div>
</div>
mohon bantuannya untuk melihat datanya terima kasih
1
1 Jawaban:
Cara kamu menampilkan gambar sekarang:
<img src="<?base_url('assets/img/pelamar/' .$profil->pas_foto_ijazah)?>" style="width:100px">
- Apakah memang gambar kamu ada di folder tersebut? /assets/img/pelamar
- Cek apakah saat die($profil->pas_foto_ijazah) sudah keluar nama file yang kamu mau?
- Cek error di console
0
Tanggapan
- Gambar sudah ada di Folder Tersebut.
- Untuk Nama file keluar random "169365286164f3177d95e49.jpg" seperti itu
error yang muncul di console apa? coba akses langsung dari URL , apakah gambarnya keluar?